Link specifications
Design your link specifications
Now that you have your service and actions specification, you can link your service.
Here are some design questions to answer:
Question | Guidance |
---|---|
Which roles will applications have when linking my service? | It's common for services to be linked for roles such as read, write, push, consume, etc. In simple scenarios, you can just handle these situations with a property, but in more complex scenarios, you'll want to implement multiple link types. Read the section for more details. |
Which properties do I need to hold for the link? | These are the properties that define the link. They are usually a combination of input parameters plus values obtained from the cloud provider upon service creation. Set these as a JSON schema in the attributes field. |
Where in the organization is the link going to be available? | A good default is to start by making the service visible everywhere with the value organization=your-org-id:account=* . Set your choice in the visible_to array. |
Multiple link types
When applications need to link your service for different uses such as "read", "write", "push", "consume", it can be smart to have several link types. By doing so you will be able to:
- Have separate forms, schemas, and parameters for each link type.
- Implement different set of actions, particularly custom actions.
When you create several link specifications for the same service, the platform will display a dropdown menu for end users to select the type of link they want to create:
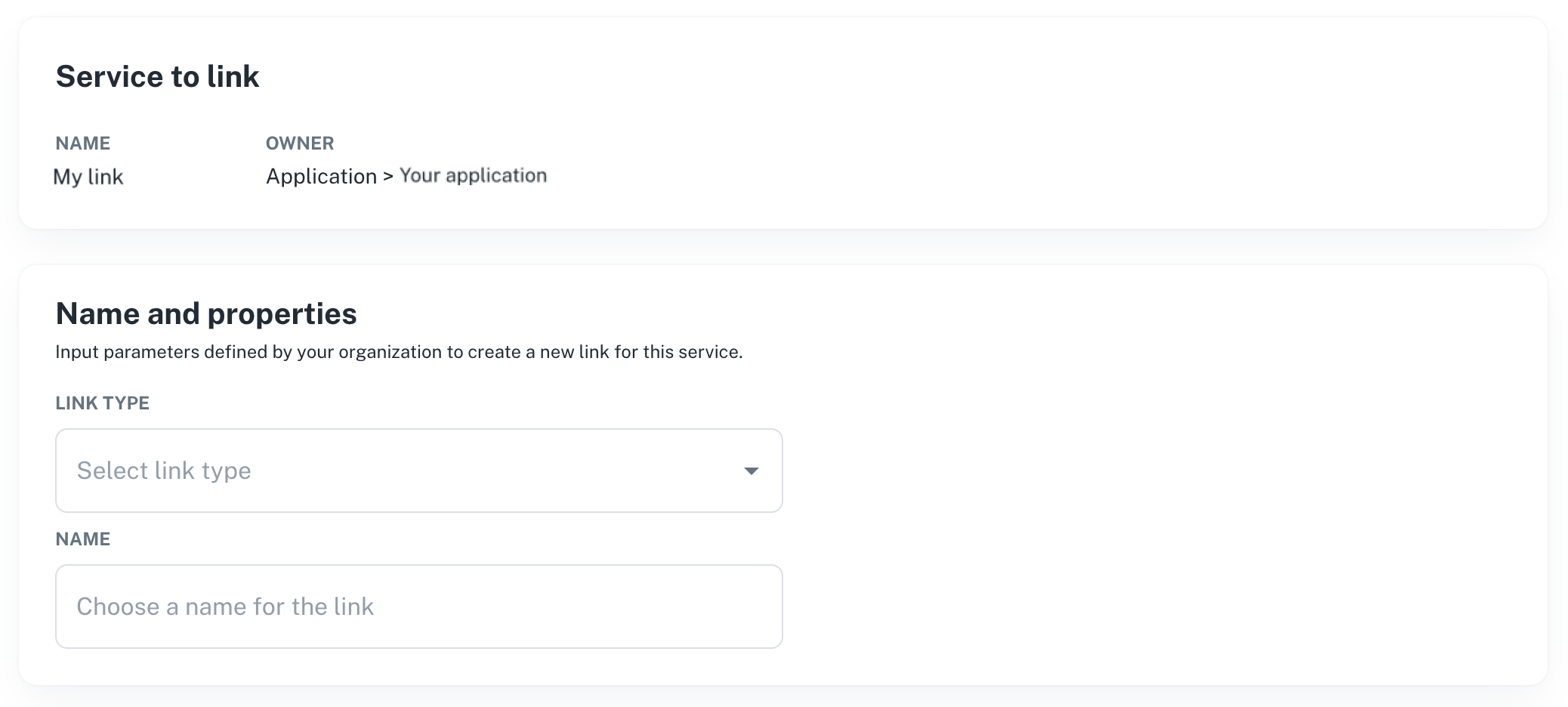
The drawback of multiple link types is that you end up with more specifications to manage, but it greatly improves UX and simplifies the provisioning code.
Create a link specification
Link specifications work very much like service specifications. Here's an example on how you should create them (see our API reference for more info):
- CLI
- cURL
np link_specification create \
--body '{
"name": "my-link-specification",
"specification_id": "fb2c761d-a3d5-19c3-58e7-72a182d49b61",
"unique": true,
"dimensions": {
"environment": "production"
},
"attributes": {
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
}
}
curl -L 'https://api.nullplatform.com/link_specification' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-d '{
"name": "my-link-specification",
"specification_id": "fb2c761d-a3d5-19c3-58e7-72a182d49b61",
"unique": true,
"dimensions": {
"environment": "production"
},
"attributes": {
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
}
}
Link action specifications
Action specifications for link work exactly the same way as they do for services. Here are examples for create, update, delete, and custom link actions (see our API reference for more info).
Create
- CLI
- cURL
np link-specification action-specification create \
--linkSpecificationId $link_spec_id \
--body '{
"name": "Create my link action spec",
"type": "create",
"parameters": { // defines the input requirements for the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"results": { // defines the expected output of the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"service_specification_id": "134544a6-a089-43ba-9d52-5b6e6e4fa8d1", // the associated service specification
"link_specification_id": "f3e8be90-0952-4506-bd54-c4e75a5a4393" // the associated link specification
}'
curl -X POST "https://api.nullplatform.com/link_specification/$link_spec_id/action_specification" \
-H "Content-Type: application/json" \
-d '{
"name": "Create my link action",
"type": "create",
"parameters": { // defines the input requirements for the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"results": { // defines the expected output of the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"service_specification_id": "134544a6-a089-43ba-9d52-5b6e6e4fa8d1", // the associated service specification
"link_specification_id": "f3e8be90-0952-4506-bd54-c4e75a5a4393" // the associated link specification
}'
Delete
- CLI
- cURL
np link-specification action-specification create \
--linkSpecificationId $link_spec_id \
--body '{
"name": "Create my link delete action spec",
"type": "delete",
"parameters": { // defines the input requirements for the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"results": { // defines the expected output of the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"service_specification_id": "134544a6-a089-43ba-9d52-5b6e6e4fa8d1", // the associated service specification
"link_specification_id": "f3e8be90-0952-4506-bd54-c4e75a5a4393" // the associated link specification
}'
curl -X POST "https://api.nullplatform.com/link_specification/$link_spec_id/action_specification" \
-H "Content-Type: application/json" \
-d '{
"name": "Create my link delete action spec",
"type": "delete",
"parameters": { // defines the input requirements for the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"results": { // defines the expected output of the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"service_specification_id": "134544a6-a089-43ba-9d52-5b6e6e4fa8d1", // the associated service specification
"link_specification_id": "f3e8be90-0952-4506-bd54-c4e75a5a4393" // the associated link specification
}'
Update
- CLI
- cURL
np link-specification action-specification create \
--linkSpecificationId $link_spec_id \
--body '{
"name": "Create my link update action spec",
"type": "update",
"parameters": { // defines the input requirements for the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"results": { // defines the expected output of the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"service_specification_id": "134544a6-a089-43ba-9d52-5b6e6e4fa8d1", // the associated service specification
"link_specification_id": "f3e8be90-0952-4506-bd54-c4e75a5a4393" // the associated link specification
}'
curl -X POST "https://api.nullplatform.com/link_specification/$link_spec_id/action_specification" \
-H "Content-Type: application/json" \
-d '{
"name": "Create my link update action spec",
"type": "update",
"parameters": { // defines the input requirements for the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"results": { // defines the expected output of the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"service_specification_id": "134544a6-a089-43ba-9d52-5b6e6e4fa8d1", // the associated service specification
"link_specification_id": "f3e8be90-0952-4506-bd54-c4e75a5a4393" // the associated link specification
}'
Custom
- CLI
- cURL
np link-specification action-specification create \
--linkSpecificationId $link_spec_id \
--body '{
"name": "Create my link custom action spec",
"type": "custom",
"parameters": { // defines the input requirements for the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"results": { // defines the expected output of the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"service_specification_id": "134544a6-a089-43ba-9d52-5b6e6e4fa8d1", // the associated service specification
"link_specification_id": "f3e8be90-0952-4506-bd54-c4e75a5a4393" // the associated link specification
}'
curl -X POST "https://api.nullplatform.com/link_specification/$link_spec_id/action_specification" \
-H "Content-Type: application/json" \
-d '{
"name": "Create my service custom action",
"type": "custom",
"parameters": { // defines the input requirements for the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"results": { // defines the expected output of the action.
"schema": {
"type": "object",
"properties": {
"my_string_property": {
"type": "string"
},
"my_number_property": {
"type": "number",
"default": 0
}
},
"required": [
"my_string_property"
]
},
"values": {}
},
"service_specification_id": "134544a6-a089-43ba-9d52-5b6e6e4fa8d1", // the associated service specification
"link_specification_id": "f3e8be90-0952-4506-bd54-c4e75a5a4393" // the associated link specification
}'