Designing the UI
By default, nullplatform will render any specification with a default renderer, but you can customize the look and feel of your services, links, and action forms through JSON UI Schema.
To create a JSON UI schema you have to add an uiSchema
element in your specifications under the schema
element.
Refer to the following sections to get some real-world scenarios and examples.
JSON UI Schema
The JSON UI schema defines the structure and layout of a form using a standard JSON object.
Below is an example of an uiSchema
object and how it appears in the UI. To make it easier to follow, we divided the
example into smaller snippets. The full JSON schema example can be found at the end of the section.
Including markdown
Click to expand the JSON example.
{
// ...service specification
"attributes": {
"schema": {
"uiSchema": {
"type": "VerticalLayout",
"elements": [
{
"type": "Group",
"label": "nullplatform supports UI schema",
"elements": [
{
"type": "Label",
"text": "\"You can create your own interfaces using *UI schema* which has full support for `markdown`\".\n\n\nAlso, you can create advanced interactions such as:\n* controls that are conditionally shown/hidden\n* tabs\n...\n* and more.\n\nCheck out the showcase of supported elements in the next group.",
"options": {
"format": "markdown"
}
}
]
}
]
},
// ...the traditional JSON schema
}
}
}
The UI schema will generate a form similar to this:
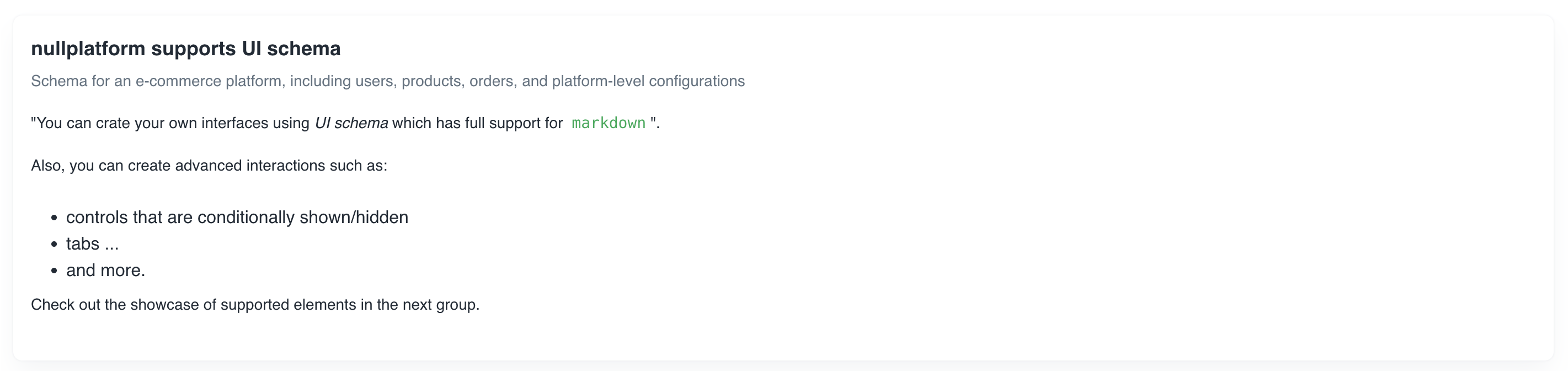
Decorating controls
Click to expand the JSON example.
{
// ...service specification
"attributes": {
"schema": {
"uiSchema": {
"type": "VerticalLayout",
"elements": [
{
"type": "Group",
"label": "Platform Configuration",
"elements": [
{
"type": "Label",
"text": "> [!info]\n> **Note:** The following settings are used to configure the e-commerce platform.",
"options": {
"format": "markdown"
}
},
{
"type": "Control",
"scope": "#/properties/platformName",
"label": "Platform Name"
},
{
"type": "Control",
"scope": "#/properties/platformVersion",
"label": "Platform Version"
},
{
"type": "Control",
"scope": "#/properties/supportEmail",
"label": "Support Email"
},
{
"type": "Control",
"scope": "#/properties/defaultCurrency",
"label": "Default Currency"
},
{
"type": "Label",
"text": "Available [coins](https://www.iban.com/exchange-rates)",
"options": {
"format": "markdown"
}
},
{
"type": "Control",
"scope": "#/properties/taxRate",
"label": "Tax Rate (%)"
},
{
"type": "Control",
"scope": "#/properties/maintenanceMode",
"label": "Maintenance Mode"
}
]
}
]
}
}
}
}
The UI schema will generate a form similar to this:
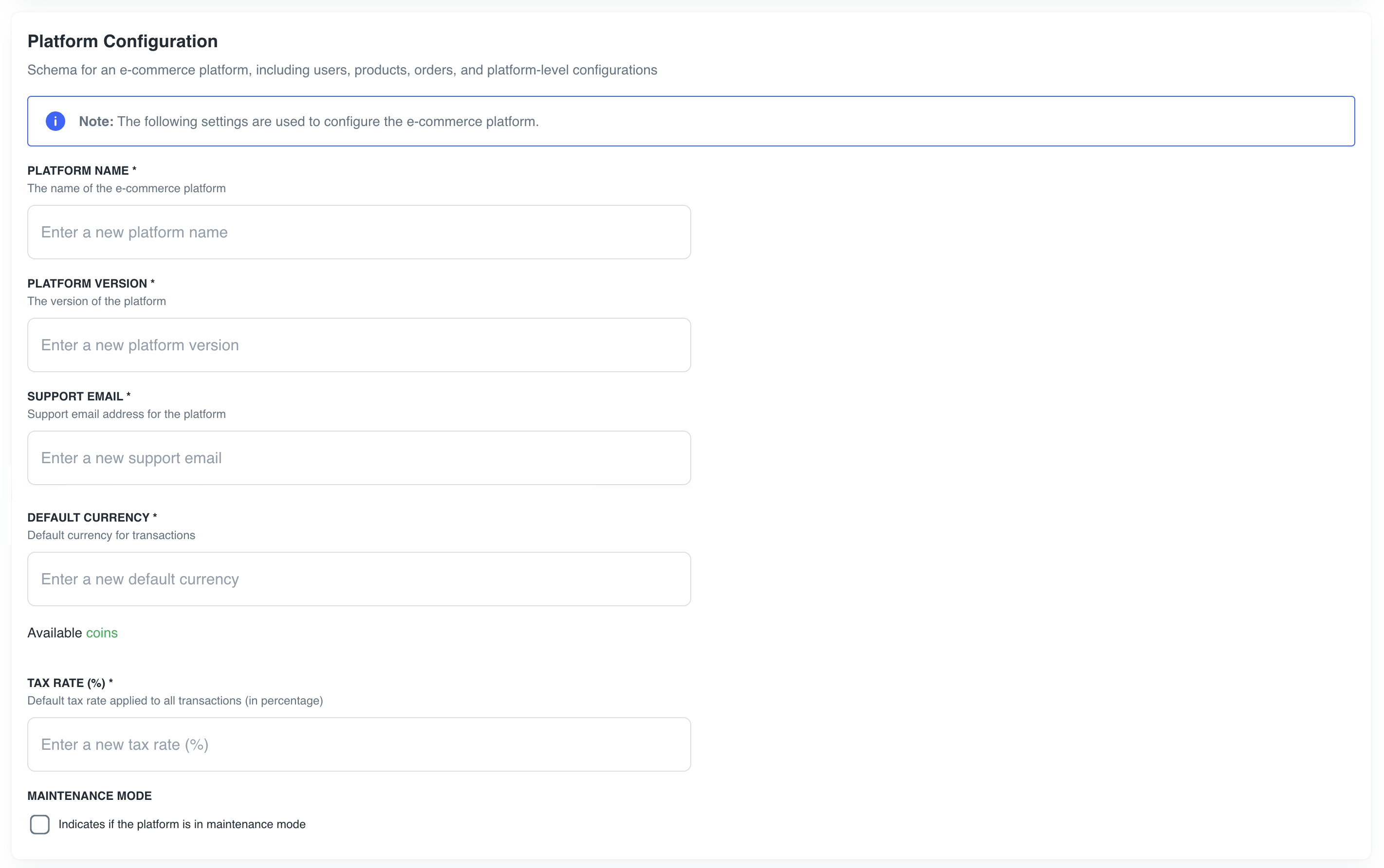
Arrays and collapsible groups
Click to expand the JSON example.
{
// ...service specification
"attributes": {
"schema": {
"uiSchema": {
"type": "VerticalLayout",
"elements": [
{
"type": "Group",
"label": "Orders",
"elements": [
{
"type": "Control",
"scope": "#/properties/orders",
"options": {
"detail": {
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/items/properties/orderId",
"label": "Order ID"
},
{
"type": "Control",
"scope": "#/items/properties/userId",
"label": "User ID"
},
{
"type": "Control",
"scope": "#/items/properties/status",
"label": "Status"
},
{
"type": "Control",
"scope": "#/items/properties/orderItems",
"options": {
"detail": {
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/items/properties/productId",
"label": "Product ID"
},
{
"type": "Control",
"scope": "#/items/properties/quantity",
"label": "Quantity"
},
{
"type": "Control",
"scope": "#/items/properties/price",
"label": "Price"
}
]
}
}
},
{
"type": "Control",
"scope": "#/items/properties/totalPrice",
"label": "Total Price"
},
{
"type": "Control",
"scope": "#/items/properties/createdAt",
"label": "Created At"
}
]
}
}
}
]
},
{
"type": "Categorization",
"elements": [
{
"type": "Category",
"label": "Orders",
"elements": [
{
"type": "Label",
"text": "### Fetch all orders\n```bash\ncurl -X POST https://api.example.com/orders -d '{\"productId\": \"1234\", \"quantity\": 2}'\n```### Update order status\n```bash\ncurl -X PUT https://api.example.com/orders/1234 -d '{\"status\": \"shipped\"}'\n```",
"options": {
"format": "markdown"
}
}
]
},
{
"type": "Category",
"label": "Users",
"elements": [
{
"type": "Label",
"text": "### Fetch all users\n```bash\ncurl -X GET https://api.example.com/users\n```### Update user details\n```bash\ncurl -X PUT https://api.example.com/users/1234 -d '{\"name\": \"John Doe\"}'\n```",
"options": {
"format": "markdown"
}
}
]
}
],
"options": {
"collapsible": {
"collapsed": true,
"label": "Add help like this"
}
}
}
]
}
// ...traditional JSON schema
}
}
}
The UI schema will generate a form similar to this:
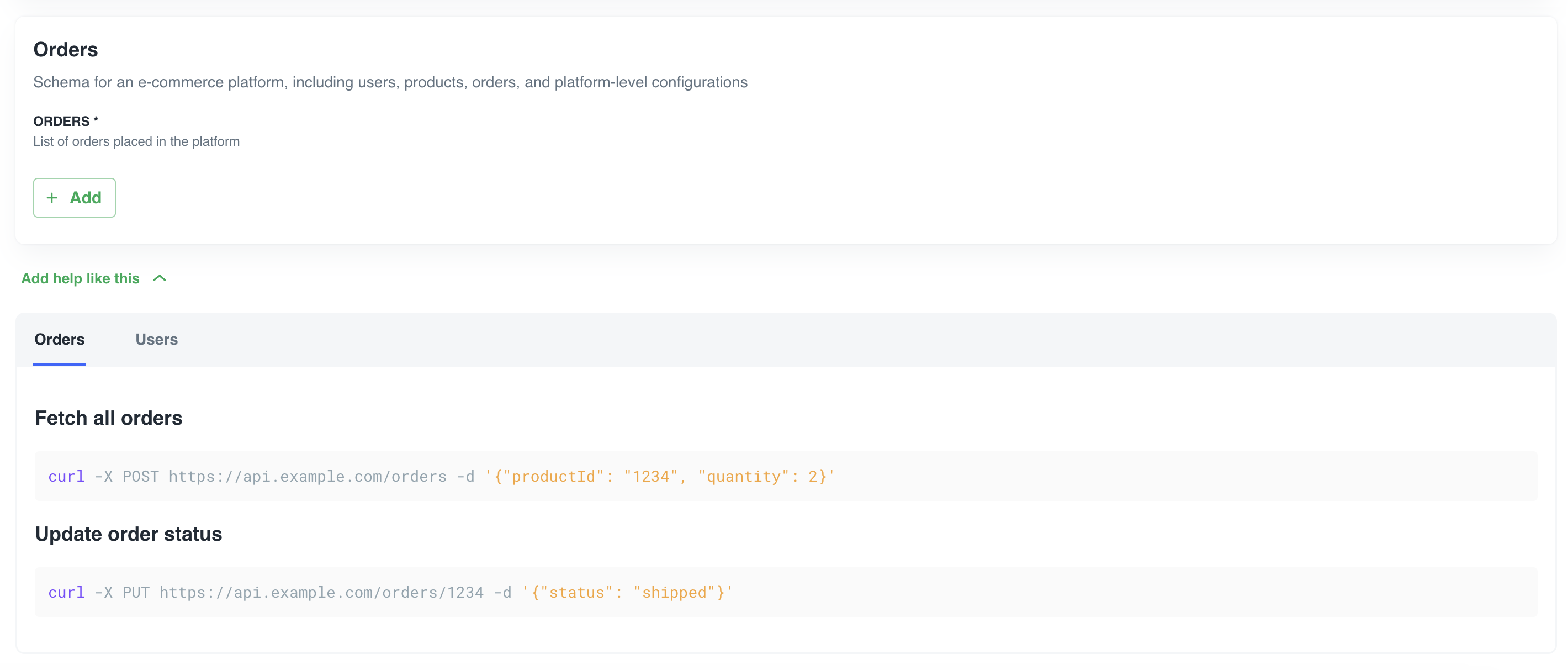
For more details, refer to the JSON UI Schema documentation.
Full example
Click to expand the complete JSON uiSchema example.
{
"title": "E-Commerce Platform Schema",
"description": "Schema for an e-commerce platform, including users, products, orders, and platform-level configurations",
"type": "object",
"properties": {
"platformName": {
"type": "string",
"description": "The name of the e-commerce platform",
"minLength": 1
},
"platformVersion": {
"type": "string",
"description": "The version of the platform",
"pattern": "^\\d+\\.\\d+\\.\\d+$"
},
"supportEmail": {
"type": "string",
"description": "Support email address for the platform",
"format": "email"
},
"defaultCurrency": {
"type": "string",
"description": "Default currency for transactions",
"pattern": "^[A-Z]{3}$",
"examples": [
"USD",
"EUR",
"GBP"
]
},
"taxRate": {
"type": "number",
"description": "Default tax rate applied to all transactions (in percentage)",
"minimum": 0,
"maximum": 100
},
"maintenanceMode": {
"type": "boolean",
"description": "Indicates if the platform is in maintenance mode",
"default": false
},
"users": {
"type": "array",
"description": "List of users in the platform",
"items": {
"type": "object",
"properties": {
"userId": {
"type": "string",
"format": "uuid"
},
"name": {
"type": "string",
"minLength": 1
},
"email": {
"type": "string",
"format": "email"
},
"createdAt": {
"type": "string",
"format": "date-time"
},
"isAdmin": {
"type": "boolean",
"default": false
}
},
"required": [
"userId",
"name",
"email",
"createdAt"
]
}
},
"products": {
"type": "array",
"description": "List of products available in the store",
"items": {
"type": "object",
"properties": {
"productId": {
"type": "string"
},
"name": {
"type": "string",
"minLength": 1
},
"description": {
"type": "string"
},
"price": {
"type": "number",
"minimum": 0
},
"stock": {
"type": "integer",
"minimum": 0
},
"category": {
"type": "string",
"enum": [
"electronics",
"clothing",
"home",
"sports",
"books"
]
},
"reviews": {
"type": "array",
"description": "User reviews for the product",
"items": {
"type": "object",
"properties": {
"reviewId": {
"type": "string"
},
"userId": {
"type": "string",
"format": "uuid"
},
"rating": {
"type": "integer",
"minimum": 1,
"maximum": 5
},
"comment": {
"type": "string",
"maxLength": 500
},
"createdAt": {
"type": "string",
"format": "date-time"
}
},
"required": [
"reviewId",
"userId",
"rating",
"createdAt"
]
}
}
},
"required": [
"productId",
"name",
"price",
"stock",
"category"
]
}
},
"orders": {
"type": "array",
"description": "List of orders placed in the platform",
"items": {
"type": "object",
"properties": {
"orderId": {
"type": "string"
},
"userId": {
"type": "string",
"format": "uuid"
},
"status": {
"type": "string",
"enum": [
"pending",
"shipped",
"delivered",
"canceled"
]
},
"orderItems": {
"type": "array",
"items": {
"type": "object",
"properties": {
"productId": {
"type": "string"
},
"quantity": {
"type": "integer",
"minimum": 1
},
"price": {
"type": "number",
"minimum": 0
}
},
"required": [
"productId",
"quantity",
"price"
]
}
},
"totalPrice": {
"type": "number",
"minimum": 0,
"description": "Automatically calculated as the sum of order items"
},
"createdAt": {
"type": "string",
"format": "date-time"
}
},
"required": [
"orderId",
"userId",
"status",
"orderItems",
"totalPrice",
"createdAt"
]
}
}
},
"required": [
"platformName",
"platformVersion",
"supportEmail",
"defaultCurrency",
"taxRate",
"users",
"products",
"orders"
],
"uiSchema": {
"type": "VerticalLayout",
"elements": [
{
"type": "Group",
"label": "nullplatform supports UI schema",
"elements": [
{
"type": "Label",
"text": "\"You can create your own interfaces using *UI schema* which has full support for `markdown`\".\n\n\nAlso, you can create advanced interactions such as:\n* controls that are conditionally shown/hidden\n* tabs\n...\n* and more.\n\nCheck out the showcase of supported elements in the next group.",
"options": {
"format": "markdown"
}
}
]
},
{
"type": "Group",
"label": "Platform Configuration",
"elements": [
{
"type": "Label",
"text": "> [!info]\n> **Note:** The following settings are used to configure the e-commerce platform.",
"options": {
"format": "markdown"
}
},
{
"type": "Control",
"scope": "#/properties/platformName",
"label": "Platform Name"
},
{
"type": "Control",
"scope": "#/properties/platformVersion",
"label": "Platform Version"
},
{
"type": "Control",
"scope": "#/properties/supportEmail",
"label": "Support Email"
},
{
"type": "Control",
"scope": "#/properties/defaultCurrency",
"label": "Default Currency"
},
{
"type": "Label",
"text": "Available [coins](https://www.iban.com/exchange-rates)",
"options": {
"format": "markdown"
}
},
{
"type": "Control",
"scope": "#/properties/taxRate",
"label": "Tax Rate (%)"
},
{
"type": "Control",
"scope": "#/properties/maintenanceMode",
"label": "Maintenance Mode"
}
]
},
{
"type": "Group",
"label": "Users",
"elements": [
{
"type": "Label",
"text": "All users registered in the platform are available [here](https://example.com/users)",
"options": {
"format": "markdown"
}
},
{
"type": "Control",
"scope": "#/properties/users",
"options": {
"detail": {
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/items/properties/userId",
"label": "User ID"
},
{
"type": "Control",
"scope": "#/items/properties/name",
"label": "Name"
},
{
"type": "Control",
"scope": "#/items/properties/email",
"label": "Email"
},
{
"type": "Control",
"scope": "#/items/properties/createdAt",
"label": "Created At"
},
{
"type": "Control",
"scope": "#/items/properties/isAdmin",
"label": "Admin"
}
]
}
}
}
]
},
{
"type": "Group",
"label": "Products",
"elements": [
{
"type": "Control",
"scope": "#/properties/products",
"options": {
"detail": {
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/items/properties/productId",
"label": "Product ID"
},
{
"type": "Control",
"scope": "#/items/properties/name",
"label": "Name"
},
{
"type": "Control",
"scope": "#/items/properties/description",
"label": "Description"
},
{
"type": "Control",
"scope": "#/items/properties/price",
"label": "Price"
},
{
"type": "Control",
"scope": "#/items/properties/stock",
"label": "Stock"
},
{
"type": "Control",
"scope": "#/items/properties/category",
"label": "Category"
},
{
"type": "Control",
"scope": "#/items/properties/reviews",
"options": {
"detail": {
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/items/properties/reviewId",
"label": "Review ID"
},
{
"type": "Control",
"scope": "#/items/properties/userId",
"label": "User ID"
},
{
"type": "Control",
"scope": "#/items/properties/rating",
"label": "Rating"
},
{
"type": "Control",
"scope": "#/items/properties/comment",
"label": "Comment"
},
{
"type": "Control",
"scope": "#/items/properties/createdAt",
"label": "Created At"
}
]
}
}
}
]
}
}
}
]
},
{
"type": "Group",
"label": "Orders",
"elements": [
{
"type": "Control",
"scope": "#/properties/orders",
"options": {
"detail": {
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/items/properties/orderId",
"label": "Order ID"
},
{
"type": "Control",
"scope": "#/items/properties/userId",
"label": "User ID"
},
{
"type": "Control",
"scope": "#/items/properties/status",
"label": "Status"
},
{
"type": "Control",
"scope": "#/items/properties/orderItems",
"options": {
"detail": {
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/items/properties/productId",
"label": "Product ID"
},
{
"type": "Control",
"scope": "#/items/properties/quantity",
"label": "Quantity"
},
{
"type": "Control",
"scope": "#/items/properties/price",
"label": "Price"
}
]
}
}
},
{
"type": "Control",
"scope": "#/items/properties/totalPrice",
"label": "Total Price"
},
{
"type": "Control",
"scope": "#/items/properties/createdAt",
"label": "Created At"
}
]
}
}
}
]
},
{
"type": "Categorization",
"elements": [
{
"type": "Category",
"label": "Orders",
"elements": [
{
"type": "Label",
"text": "### Fetch all orders\n```bash\ncurl -X POST https://api.example.com/orders -d '{\"productId\": \"1234\", \"quantity\": 2}'\n```### Update order status\n```bash\ncurl -X PUT https://api.example.com/orders/1234 -d '{\"status\": \"shipped\"}'\n```",
"options": {
"format": "markdown"
}
}
]
},
{
"type": "Category",
"label": "Users",
"elements": [
{
"type": "Label",
"text": "### Fetch all users\n```bash\ncurl -X GET https://api.example.com/users\n```### Update user details\n```bash\ncurl -X PUT https://api.example.com/users/1234 -d '{\"name\": \"John Doe\"}'\n```",
"options": {
"format": "markdown"
}
}
]
}
],
"options": {
"collapsible": {
"collapsed": true,
"label": "Add help like this"
}
}
}
]
}
}